Working with vCloud Automation Center IaaS entities
vCloud Automation Center has several constructs such as blueprints, business groups, reservations to manage the virtual machines lifecycle and governance policies.
While most customers will use vCloud Automation Center out of the box some have several use cases for automation and integration. This is where vCenter Orchestrator comes into the picture.
The vCloud Automation Center Infrastructure as a service data model (vCAC IaaS)
vCAC IaaS has an extensible model consisting of different metamodels. Here are some of the meta models:
- ManagementModelEntities.svc: Generic model providing an abstraction layer to different vendor endpoints.
Which is extended by specific models:
- AmazonWSModelEntities.svc : To manage Amazon Web service
- ScvmmModelEntities.svc : To manage Microsoft System Center Virtual Machine Manager
- UcsModelEntities.svc :To manage Cisco UCS Manager
A new model can be created to extend the management capabilities. Each model has a number of entity sets. Here are a few of the ManagementModelEntities model entity sets:
- VirtualMachineTemplates : The vCAC blueprints (abstracting several vendors virtual machine / vApp templates).
- VirtualMachineTemplateProperties: The vCAC blueprint custom properties
- VirtualMachines : The vCAC VMs deployed from the blueprints
- VirtualMachineProperties : The vCAC blueprint custom properties
Each entity set contains a list of entities, For example the list of all the blueprints that have been created (VirtualMachineTemplates). Each entity has a list a properties (i.e ID, name and so on) and a list of linked entities. The image below shows some entities, their properties and how they are linked together:
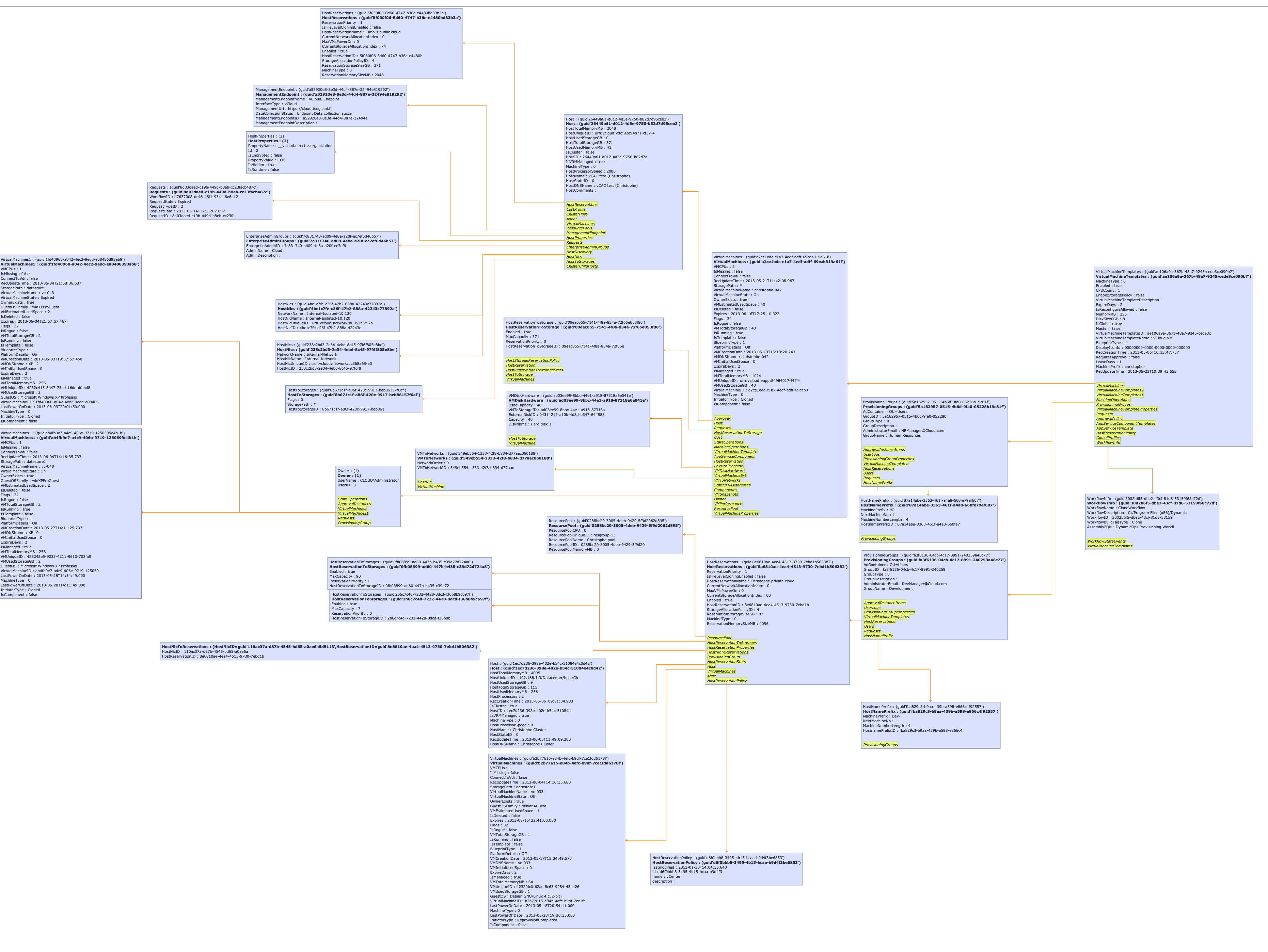
Accessing to the data model through the API
Since IaaS is build using Microsoft .net it leverages the Open Data Protocol (also called OData). You can find more about OData here.
Odata basically exposes the data model as a REST API. If you use the URL of one of the management model you can browse the entities as XML atom files. JSON is also supported.
The good think about using the OData API is that you can access all entities in the data model, it is quite powerfull. The issue may be that with great power comes great responsibility. The data model changed between vCAC IaaS versions. These changes may break your API usage. Also if you do unexpected changes in the data model you may break some of the IaaS functionality and loose product support. For example information panels on the IaaS web site do break when some entities are missing links to other entities that are expected. The OData API is not a public API and will eventually be abstracted by a higher level service catalog API and provide the functionality required for most of the automation / integration use cases.
Automation and integration use cases
There are quite a few reasons you may need the vCAC IaaS API such as:
- Having your own custom portal to leverage the vCAC capabilities
- Automating some of the tasks that would require a vCAC admin to do several times the same operation in the user interface. For example creating hundreds of provisioning groups.
- In some cases when vCAC is calling out to a sub system, the sub system may need to call back vCAC to get further information or to update some vCAC entities. For example adding a custom property on a vCAC VM with an external ticketing system request ID.
Automating and integrating vCAC IaaS using the vCAC Iaas plug-in for vCenter Orchestrator
Combine vCAC IaaS and vCenter Orchestrator and you have the best combination of policy based lifecycle management and technical orchestration. vCAC IaaS has a vCO endpoint allowing to run vCO workflows either as part of the IaaS lifecycle steps or with extending second day operations on VMs.
vCenter Orchestrator has a vCAC IaaS plug-in allowing to automate vCAC. You can find this plug-in here. The plug-in includes the “vCAC extensibility package” which allows to extend vCAC IaaS in a few clicks with vCenter Orchestrator workflows. You can find more information about the) extensibility package here. The plug-in is providing several facilities including :
- An inventory of objects allowing end users to select items as a tree view or list:
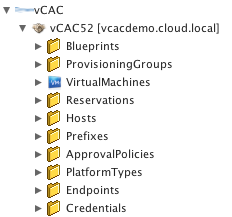
-
A set of workflows for:
-
Configuring vCAC hosts
-
Performing CRUD operations on credential, endpoints, enterprise groups, machine prefixes and provisioning groups
-
Register VMs to be managed by vCAC IaaS
-
Use a vCO workflow as a vCAC IaaS menu operation or to extend the IaaS VM lifecycle
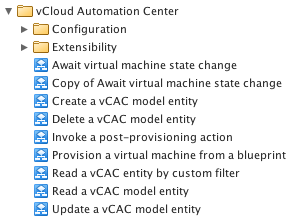
The rest of the workflow listed above is mainly to get, create, update delete model entities.
Working with model entities
If you want to do anything on the entities that are not managed by the workflows in the administration folder then you will need to work with the entity object.
Getting a model entity using an inventory object
You may wonder how do you start. If the entity you are looking for has an object listed in the vCO inventory you can just use an input parameter of the given type. For example I have created a workflow with an input of vCAC:BluePrint type. Once I run the workflow, as an end user I can select a blueprint by name but also based on several other properties of the blueprint.
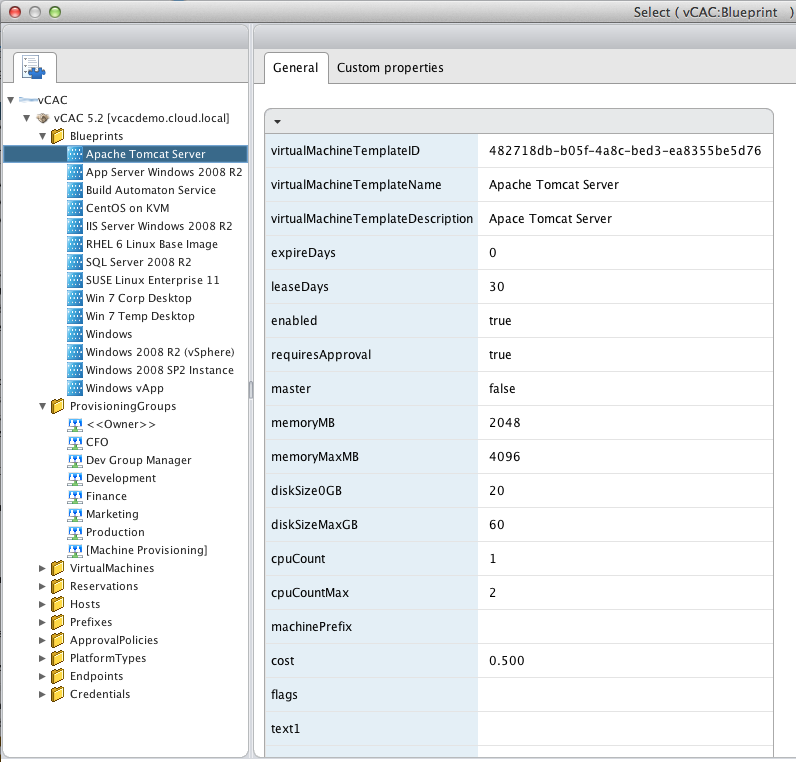
You can access to the properties displayed in the inventory and additional ones by scripting.
The API Explorer lists the following properties and methods:
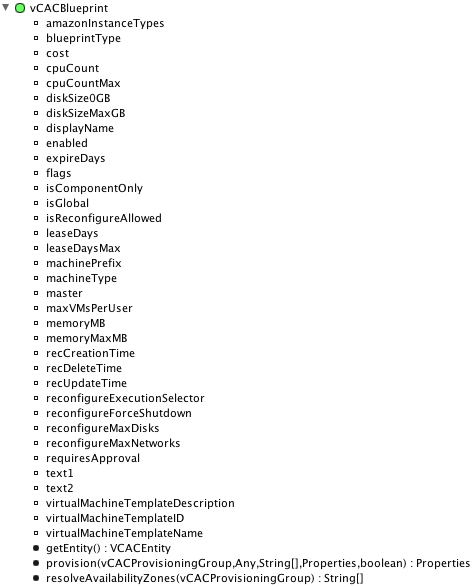
So for example blueprint.memoryMB will return 2048.
This allows to read the blueprint properties. To modifiy the blueprint properties or to get the blueprint linked entities requires to get the entity object backing up this inventory object.
|
|
In a few cases the inventory object is not the same as the entity set name. For example the code above will first print “VirtualMachineTemplates” for a blueprint. VirtualMachineTemplate is how blueprints are called in the data model, “blueprint” is the name used in the end user interface and documentation. Since the inventory objects are aimed at end users it matches the user interface one.
The last line will print all the available properties of the entity. This is useful whan you want to filter on a particular property to get an entity.
Getting a model entity object using filtering
You may want to get a particular entity without user intervention. For this you can use the vCACEntityManager.readModelEntitiesByCustomFilter(); For this you need to specifiy as parameters:
- The vCAC IaaS host id in which to make the search
- The model name: Here we use ManagementModelEntities.svc which contains the virtualMachineTemplates entity set.
- The entity set: Here virtualMachineTemplates.
- The properties used for filtering : Here the only property we want to filter on is the blueprint name.
- VirtualMachineTemplateName : We could have filtered on any other of the properties.
|
|
The method above will run a query on the server side. This is a lot more performant than for example pulling all the blueprints in the scripting and iterating through their properties.
Note that readModelEntitiesByCustomFilter will always return an array of elements assuming there will be 0 to n entities matching the supplied property.
Another example is to get the virtualMachine entity from a virtualMachineId. This is typically what is needed when calling a vCO workflow from a vCAC workflow stub.
|
|
You can also perform more complex queries. The code below return the top ten results of all virtual machines, filtered by the machine state and component flag
|
|
Using the entities
You will need to use the entities to get to its linked entities. For example the code below gets the virtualMachineTemplateProperties entity which matches the propertyName.
|
|
For updating this property entity you would use:
|
|
For creating a new entity property you would replace the last paragraph by:
|
|
The code above is an extract of the addUpdatePropertyFromBlueprint action that is included in the 5.2 version of the vCAC plug-in for vCO. There is a similar action called addUpdatePropertyFromVirtualMachineEntity for handling virtual machine properties.
Converting the entities back to an inventory object
If you found a particular entity usng the scripting you may want to get the inventory object it matches. In this case you can use the following method:
|
|
This of course only works for entities having a matching inventory object.
For example here is an action returning blueprints with a given name:
|
|
More information
The Using the vCenter Orchestrator plug-in for vCloud Automation Center 5.2 guide from VMware technical publications provides additional information and scripting examples.